PROCJAM 2024
PROCJAM—the procedural generation (Procgen) jam where people make things that make things—is back for its 2024 edition. I set some time aside this week to figure out whether there is any Procgen potential in Tangram—a puzzle game from 18th-century China.
I rediscovered a wooden Tangram set last year, when I helped my parents declutter and move, and instantly remembered how fascinated I was by this unimposing toy as a kid. Today I understand that this is the same kind of fascination that brought me to Procgen: Systems with small rule sets that can produce very complex outputs spark joy and my urge to explore. (Which, coincidentally, is also the reason why current “AI” and large language models do not impress me at all: complex things done by complex systems is just not that jaw-dropping.)
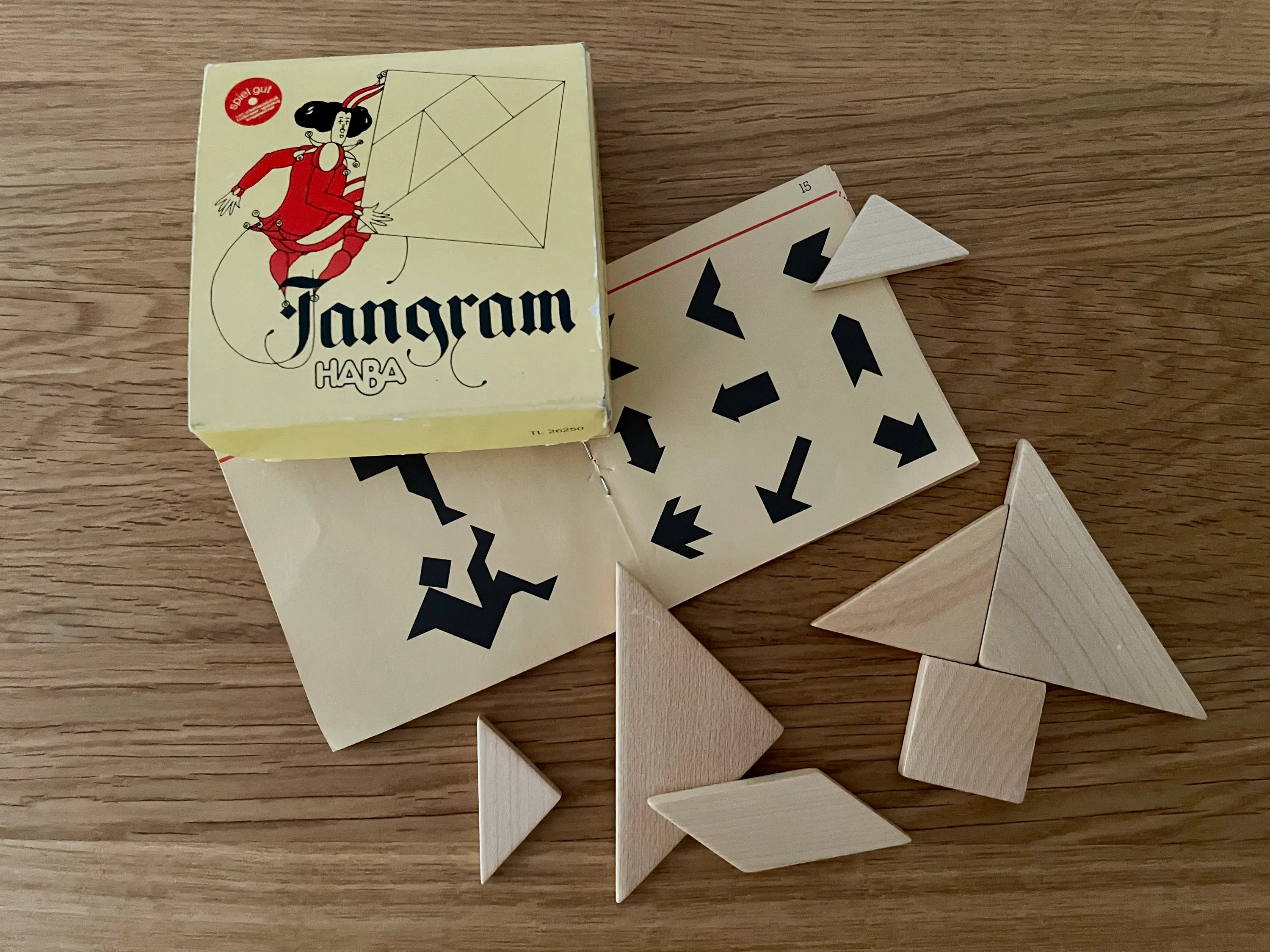
The goal of the puzzle is to replicate an outlined shape using all seven pieces, also called tans, without overlap.
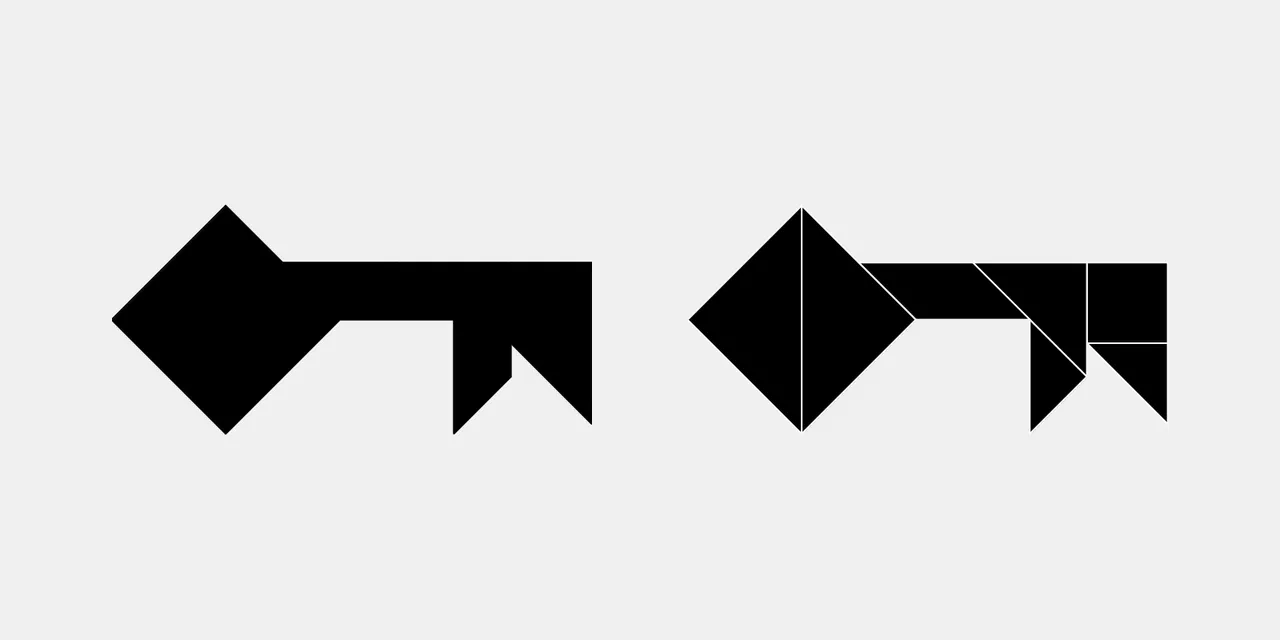
A couple of questions that popped into my head when I started thinking about Tangram’s potential for this game jam:
- How would I model and code this puzzle?
- How can I generate new puzzles?
- Given certain restricting rules, can I systematically produce all possible configurations?
- Can I experimentally prove that there are only 13 convex configurations?
- I want to create aesthetically pleasing configurations. How would I go about that?
- Are there ways to create interesting level architectures with tangram pieces? Random walker that exclusively uses valid placement of tans?
I am convinced: There is something to gain from modeling this puzzle game and casting it in code.
2024-10-26: Doodling and Hard-Coded Configurations
My first task was to model tans, be able to draw tans to the screen, and create hard-coded (as in: place tans with set coordinates on the screen) configurations. In this explorative phase, I am using the p5.js web editor and you can tinker with my code.
I think we need the following information to properly describe a tan:
- Set of vertices
- Position
- Rotation
- Reflection flag
That way we can translate, rotate, and reflect. The latter is only needed for the parallelogram, as this is the only piece without reflection symmetry. We are able to flip the wooden piece around in real life, so we need to be able to model that in code as well. All the other tans are axisymmetric, i.e. reflection or “flipping around” can be modeled by rotation. The set of vertices allows me to draw the tan onto the screen.
Side note: My Remarkable 2 has proven to be an indispensable tool in this early stage of the project; allowing me to scribble, turn handwritten into typed text, and move and rotate selected areas of the page helped me a lot wrapping my head around the linear algebra of the matter.
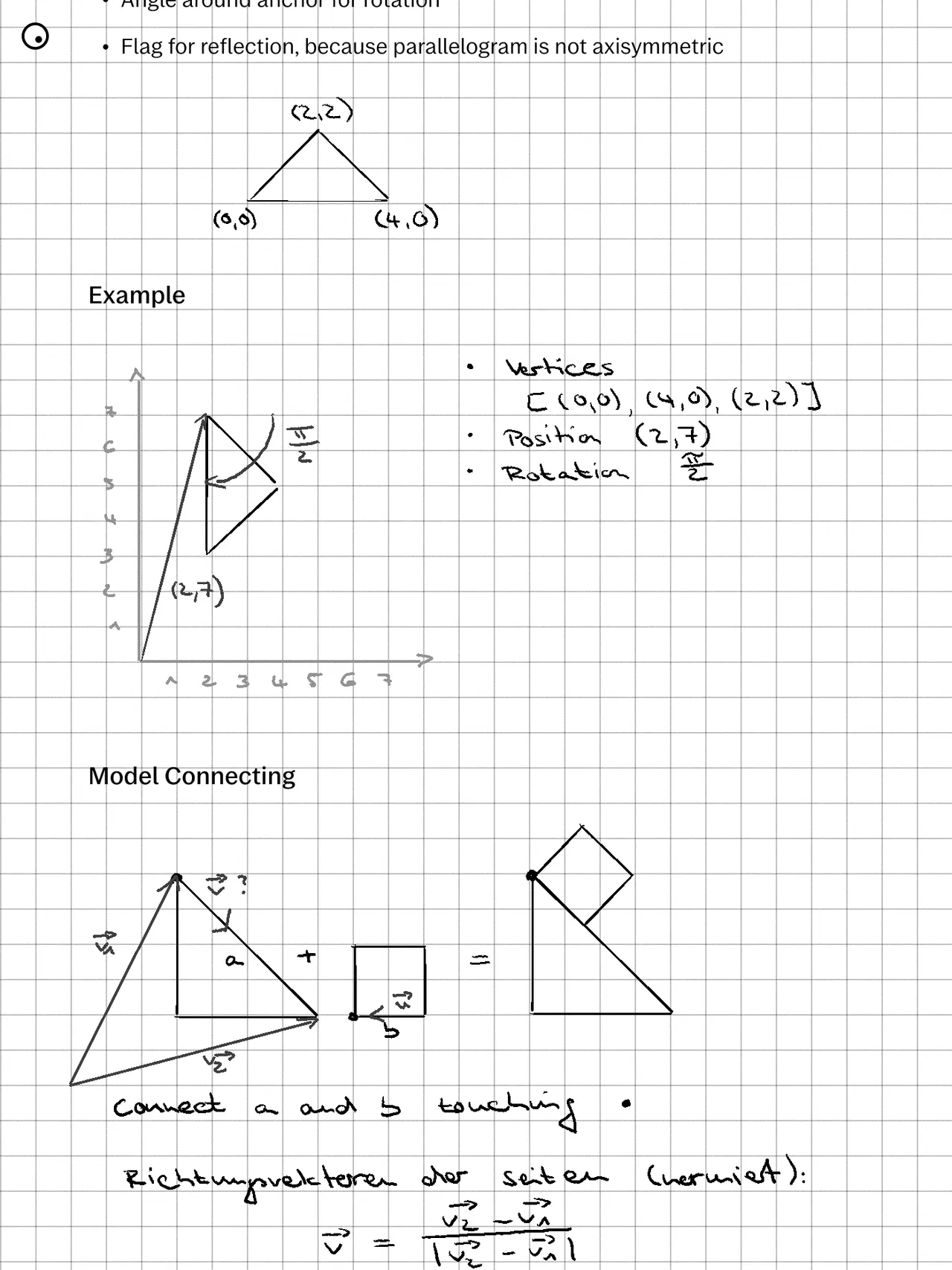
Speaking of linear algebra: It was of major value to start thinking about vertices, sides, and directions as vectors. Everything in Tangram is a vector! The first vertex of every tan is the two-dimensional zero vector (0, 0)
which serves as an anchor and rotation point for the whole shape. I set the square tan as the baseline for size with a side length of 1. That way the large triangle below would consist of the vertices [(0, 0), (2√2, 0), (√2, √2)]
. Do not forget that when dealing with computer graphics the y-axis increases downwards and p5.js is no exception.
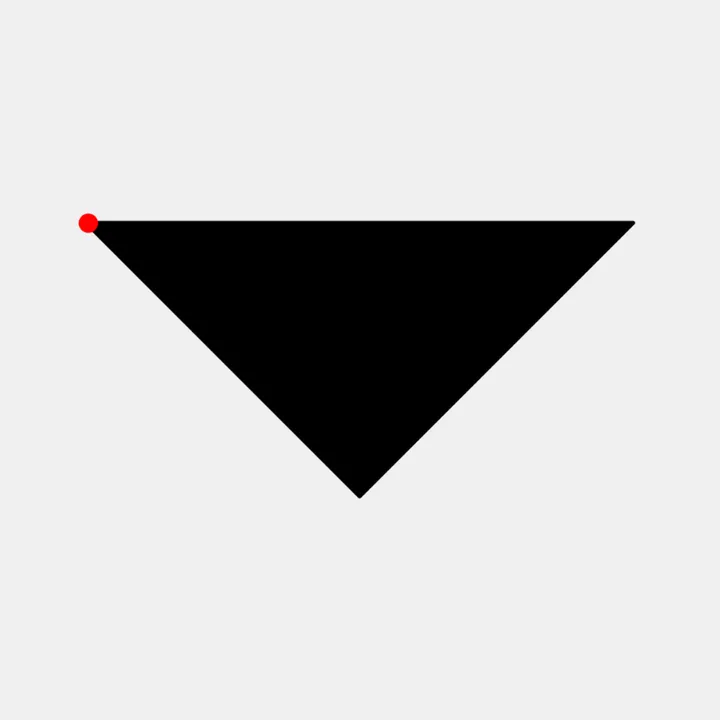
And with that we can draw tans and configurations. The code below…
TTL1.position = createVector(1, -2);
TTL1.draw();
TTL2.position = createVector(1, -2);
TTL2.rotation = QUARTER_PI;
TTL2.draw();
TTM.position = createVector(1, -2);
TTM.rotation = HALF_PI;
TTM.draw();
TTS1.position = createVector(0, -1);
TTS1.rotation = -HALF_PI;
TTS1.draw();
TS.position = createVector(0, -1 - SQRT2);
TS.rotation = -QUARTER_PI;
TS.draw();
TP.position = createVector(0.5 * SQRT2, -1 - 1.5 * SQRT2);
TP.rotation = -HALF_PI;
TP.draw();
TTS2.position = createVector(0.5 * SQRT2, -2.5 * SQRT2);
TTS2.rotation = -HALF_PI - QUARTER_PI;
TTS2.draw();
…creates this “Swan” configuration.
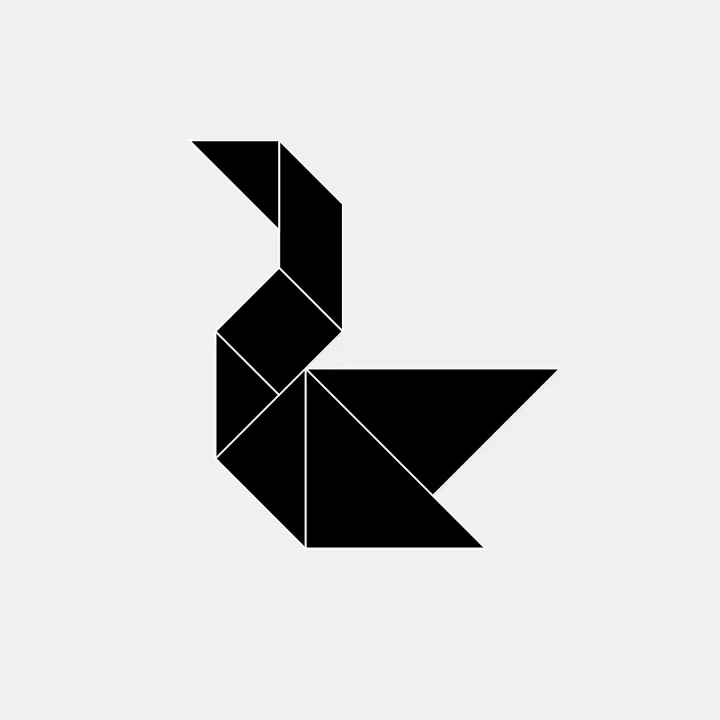
By the way, variable names like TTL1
will come up again. I am using the naming convention T
for tan, then the first letter of the shape, then size, and a number. So TTL1
stands for tan triangle large one, or the first large triangle piece. TS
would be the square, and so on.